Example 1: WebCam access with OpenCV
Introduction
In this example, we are using the PythonImage
module and access your WebCam to show the video in a View2D
.
Steps to do
Creating the network to be used for testing
Add the modules to your workspace and connect them as seen below.
The viewer is empty because the image needs to be set via Python scripting.
PythonImage
module can be found
hereCreate a macro module
Now you need to create a macro module from your network. You can either group your modules, create a local macro and convert it to a global macro module, or you use the Project Wizard and load your *.mlab file.
Add the View2D to your UI
Next, we need to add the View2D
to a Window of your macro module. Right click on your module
, open the context menu and select [
Related Files
→
<YOUR_MODULE_NAME>.script
]. The text editor
MATE
opens. You can see the *.script file of your module.
Add the following to your file:
<YOUR_MODULE_NAME>.script
Interface {
Inputs {}
Outputs {}
Parameters {}
}
Commands {
source = $(LOCAL)/<YOUR_MODULE_NAME>.py
}
Window {
h = 500
w = 500
initCommand = setupInterface
destroyedCommand = releaseCamera
Vertical {
Horizontal {
Button {
title = Start
command = startCapture
}
Button {
title = Pause
command = stopCapture
}
}
Horizontal {
expandX = True
expandY = True
Viewer View2D.self {
type = SoRenderArea
}
}
}
}
Now open the Python file of your module and define the commands to be called from the *.script file:
<YOUR_MODULE_NAME>.py
# from mevis import *
# Setup the interface for PythonImage module
def setupInterface():
pass
# Release camera in the end
def releaseCamera(_):
pass
# Start capturing WebCam
def startCapture():
pass
# Stop capturing WebCam
def stopCapture():
pass
Use OpenCV
Your View2D
is still empty, lets get access to the WebCam and show the video in your module. Open the Python file of your network again and enter the following code:
<YOUR_MODULE_NAME>.py
# from mevis import *
import cv2
import OpenCVUtils
_interfaces = []
camera = None
# Setup the interface for PythonImage module
def setupInterface():
global _interfaces
_interfaces = []
interface = ctx.module("PythonImage").call("getInterface")
_interfaces.append(interface)
# Release camera in the end
def releaseCamera(_):
pass
# Start capturing WebCam
def startCapture():
pass
# Stop capturing WebCam
def stopCapture():
pass
We now imported cv2 and OpenCVUtils so that we can use them in Python. Additionally we defined a list of _interfaces and a camera. The import of mevis is not necessary for this example.
The setupInterfaces function is called whenever the Window of your module is opened. Here we are getting the interface of the PythonImage
module and append it to our global list.
Access the WebCam
Now we want to start capturing the camera.
<YOUR_MODULE_NAME>.py
# Start capturing WebCam
def startCapture():
global camera
if not camera:
camera = cv2.VideoCapture(0)
ctx.callWithInterval(0.1, grabImage)
# Grab image from camera and update
def grabImage():
_, img = camera.read()
updateImage(img)
# Update image in interface
def updateImage(image):
_interfaces[0].setImage(OpenCVUtils.convertImageToML(image), minMaxValues = [0,255])
The startCapture function gets the camera from the cv2 object if not already available. Then it calls the current MeVisLab network context and creates a timer which calls a grabImage function every 0.1 seconds.
The grabImage function reads an image from the camera and calls updateImage. The interface from the PythonImage
module is used to set the image from the WebCam. The MeVisLab OpenCVUtils convert the OpenCV image to the MeVisLab image format MLImage.
Next, we define what happens if you click the Pause button.
<YOUR_MODULE_NAME>.py
...
# Stop capturing WebCam
def stopCapture():
ctx.removeTimers()
...
As we started a timer in our network context which updates the image every 0.1 seconds, we just stop this timer and the camera is paused.
In the end, we need to release the camera whenever you close the Window of your macro module.
<YOUR_MODULE_NAME>.py
...
# Release camera in the end
def releaseCamera(_):
global camera, _interfaces
ctx.removeTimers()
_interfaces = []
if camera:
camera.release()
camera = None
...
Again, the timers are removed, all interfaces are reset and the camera is released. The light indicating WebCam usage should turn off.
Opening your macro module via double-click
should now allow to start and pause your WebCam video in MeVisLab. You can modify your internal network using a
Convolution
filter module or any other module available in MeVisLab for modifying the stream on the fly.
Summary
- The
PythonImage
module allows to use Python for defining the image output - OpenCV can be used in MeVisLab via Python scripting
- Images and videos from OpenCV can be used in MeVisLab networks
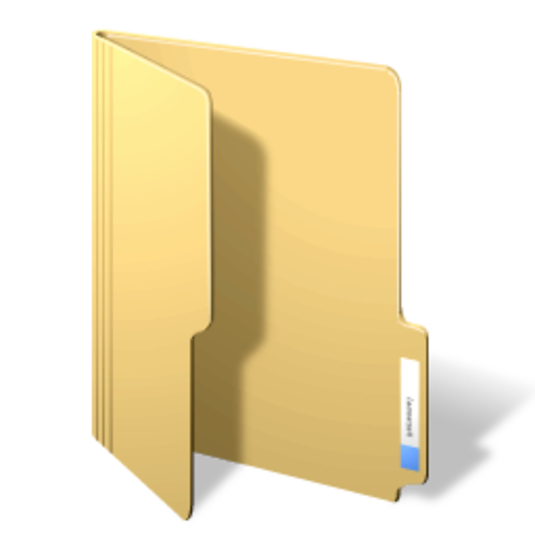