Example 2.4: Building a Panel Layout: Interactions with macro modules
Introduction
This chapter will give you an introduction into the creation of module panels and user interfaces. For the implementation you will need to use the MeVisLab Definition Language (MDL) .
Creating a panel for the macro module flilter
Creation of a module panel
In Example 2.2 we created the global macro module Filter
. By now, this module does not have a proper panel. When double-clicking
the module, the Automatic Panel is shown.
The Automatic Panel contains fields, as well as module in and outputs. In this case, no fields exists except the instanceName. Accordingly, there is no possibility to interact with the module. Only the input and the output of the module are given.
To add and edit a panel, open the context menu and select [ Related Files → Filter.script ]. The text editor MATE opens. You can see the file Filter.script, which you can edit to define a custom User Interface for the Module.
Module interface
Per default, the *.script file contains the interface of the module. In the interface section (everything insight the curled brackets behind the name Interface) you can define the module inputs, the module outputs and also all module fields (or Parameters).
Filter.script
Interface {
Inputs {
Field input0 {
internalName = Convolution.input0
}
}
Outputs {
Field output0 {
internalName = Arithmetic2.output0
}
}
}
Module inputs and outputs
To create an input/output, you need to define a Field in the respective input/output environment. Each input/output gets a name (here input0/output0) which you can use to reference this field. The module input maps to an input of the internal network. You need to define this mapping. In this case the input of the macro module Filter
maps to the input of the module Convolution
of the internal network (internalName = Convolution.input0). Similarly, you need to define which output of the internal network maps to the output of the macro module Filter
. In this example, the output of the internal module Arithmethic2
maps to the output of our macro module Filter
(internalName = Arithmetic2.output0).
Creating an input/output causes:
- Input/output connectors are added to the module.
- You can find placeholders for the input and output in the internal network (see image).
- Input/output fields are added to the automatic panel.
- A description of the input/output fields is automatically added to the module help file, when opening the *.mhelp file after input/output creation. Helpfile creation is explained in Example 2.3.
Module fields
In the environment Parameters you can define fields of your macro module. These fields may map to existing fields of the internal network (internalName = … ), but they do not need to and can also be completely new. You can reference these fields when creating a panel, to allow interactions with these fields. All fields appear in the Automatic Panel.
Module panel layout
To create your own User Interface, we need to create a Window . A window is one of the layout elements which exist in MDL. These layout elements are called controls . The curled brackets define the window environment, in which you can define properties of the window and insert further controls like a Box .
Initially, we call the window MyWindowTitle, which can be used to reference this window.
Double-clicking
on your module now opens your first self developed User Interface.
Filter.script
Interface {
Inputs {
Field input0 {
internalName = Convolution.input0
}
}
Outputs {
Field output0 {
internalName = Arithmetic2.output0
}
}
Parameters {
}
}
Window MyWindowName {
title = MyWindowTitle
Box MyBox {
}
}
You can define different properties of your control. For a window, you can for example define a title, or whether the window should be shown in full screen (fullscreen = True).
These properties are called tags and are individually different for each control. Which tags exist for the control window can be found here . The control box has different tags. You can for example define a title for the box, but you can not define whether to present the box in full screen.
If you like to add more than one control to your window, for example one box and one label, you can specify their design like in the following examples:
Filter.script
Window MyWindowName {
title = MyWindowTitle
w = 100
h = 50
Vertical {
Box MyBox {
title = "Title of my Box"
}
Label MyLabel {
title = "This is a label below the box"
}
}
}
Filter.script
Window MyWindowName {
title = MyWindowTitle
w = 100
h = 50
Horizontal {
Box MyBox {
title = "Title of my Box"
}
Label MyLabel {
title = "This is a label below the box"
}
}
}
There are much more controls, which can be used. For example a CheckBox, a Table, a Grid, a Button, ... . To find out more, take a look into the MDL Reference .
Module interactions
Until now, we learned how to create the layout of a panel. As a next step, we like to get an overview over interactions.
GUIExample
to your workspace and play around with is.Access to existing fields of the internal network
To interact with fields of the internal network in your User Interface, we
need to access these fields. To access the field of the internal module
Convolution
, which defines the kernel, we need to use the internal
network name. To find the internal field name, open the internal network of the macro module Filter
(click on the module using the middle mouse button
).
Then, open the panel of the module Convolution
and right-click
the field title Use of the box Predefined Kernel and select Copy Name. You now copied the internal network name of the field to your clipboard. The name is made up of ModuleName.FieldName, in this case Convolution.predefKernel.
In the panel of the module Convolution
, you can change this variable Kernel via a drop-down menu. In
MDL a drop-down menu is called a
ComboBox
. We can take over the field predefKernel, its drop-down menu and all its properties by
creating a new field in our panel and reference to the internal
field Convolution.predefKernel, which already exist in the internal network.
Changes of the properties of this field can be done in the curled brackets using tags (here, we changed the title).
Filter.script
Window MyWindowName {
title = MyWindowTitle
Field Convolution.predefKernel {
title = Kernel
}
}
As an alternative, you can define the field kernel in the Parameters environment, and reference the defined field by its name. The result in the panel is the same. You can see a difference in the Automatic Panel. All fields, which are defined in the interface in the Parameters environment appear in the Automatic Panel. Fields of the internal network, which are used but not declared in the section Parameters of the module interface do not appear in the Automatic Panel.
Filter.script
Interface {
Inputs {
Field input0 {
internalName = Convolution.input0
}
}
Outputs {
Field output0 {
internalName = Arithmetic2.output0
}
}
Parameters {
Field kernel {
internalName = Convolution.predefKernel
title = Kernel:
}
}
}
Window MyWindowName {
title = MyWindowTitle
Field kernel {}
}
Commands
We can not only use existing functionalities, but also add new interactions via Python scripting.
In below example we added a wakeupCommand to the Window and a simple command to the Button.
Filter.script
Window MyWindowName {
title = MyWindowTitle
wakeupCommand = myWindowCommand
Button MyButton {
command = myButtonAction
}
}
The wakeupCommand defines a Python function which is executed as soon as the Window is opened. The Button command is executed when the user clicks on the Button.
Both commands reference a Python function which is executed whenever both actions (open the Window or click the Button) are executed.
If you like to learn more about Python scripting, take a look at Example 2.5.
We need to define the Python script, which contains our Python functions. In order to do this, add a Command section outside your window and define the tag source.
Example:
Filter.script
Commands {
source = $(LOCAL)/Filter.py
}
You can right-click
on the command (myWindowCommand or myButtonAction) in your *.script file and select [
Create Python Funtion......
]. The text editor MATE opens automatically and generates an initial Python function for you. You can simply add a logging function or implement complex logic here.
Example:
Filter.py
def myWindowCommand:
MLAB.log("Window opened")
def myButtonAction:
MLAB.log("Button clicked")
Available examples
MeVisLab provides a lot of example modules for GUI development. All of these examples provides the *.script file for UI development and the *.py file containing the Python script.
Layouting examples
- TestVerticalLayout Module
- TestHorizontalLayout Module
- TestTableLayout Module
- TestGridLayout Module
- TestSplitterLayout Module
- TestBoxLayout Module
- TestTabViewLayout Module
Other examples
- TestHyperText Module
- TestListBox Module
- TestListView Module
- TestIconView Module
- TestPopupMenu Module
- TestViewers Module
- TestEventFilter Module
- TestStyles Module
- TestButtonGroups Module
- TestImageMap Module
Please use the Module Search with the prefix Test for more examples.
Summary
- User interfaces and several module panels can be created for each macro module.
- You can create a panel, define inputs and outputs as well as interactions, in your *.script file in MATE by using the MeVisLab Definition Language (MDL) .
- Module interactions can be implemented using commands, which are linked to Python functions.
- You can implement field listeners, which trigger actions after a field value changes.
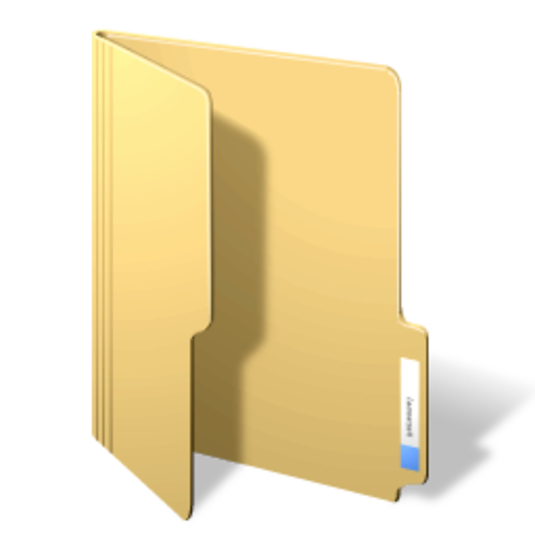